Building Scalable E-commerce Solutions with Node js and MongoDB
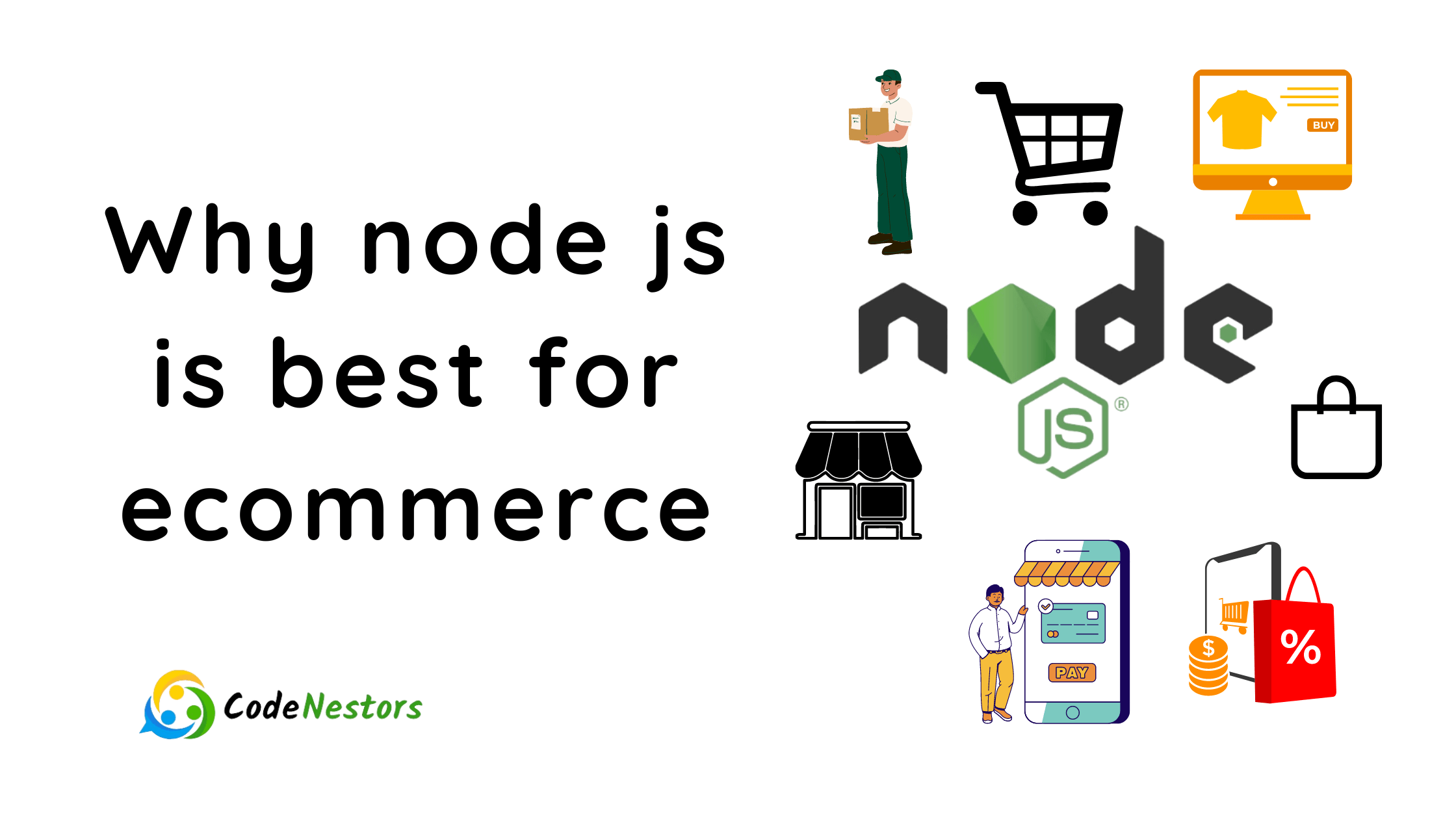
Introduction
In the fast-paced world of e-commerce, building scalable node js ecommerce solutions is crucial for handling growing user bases, managing large datasets, and ensuring a seamless shopping experience. Node js, with its asynchronous, event-driven architecture, and MongoDB, a NoSQL database known for its flexibility and scalability, make for a powerful combination in developing robust e-commerce applications. In this blog, we’ll explore the advantages and disadvantages of using Node js and MongoDB for building scalable e-commerce solutions, along with examples to illustrate key concepts.
Node js: A Brief Overview
Node js is an open-source, cross-platform JavaScript runtime that allows developers to build scalable and high-performance server-side applications. Its event-driven, non-blocking I/O model makes it ideal for handling concurrent connections and handling requests efficiently.
Unlock the Potential of Node js ECommerce
Node.js is renowned for its efficiency and speed, which are essential for e-commerce platforms that handle a multitude of user requests and transactions simultaneously. Leveraging an asynchronous, event-driven architecture, Node.js ensures that your e-commerce site can support heavy traffic without a hitch. It’s built on JavaScript, which means you can have a unified language for both server-side and client-side scripting, streamlining your development process.
Seamless Integration with MongoDB
Pairing Node.js with MongoDB, a NoSQL database, gives your e-commerce platform the flexibility to handle large volumes of data and complex transactions effortlessly. MongoDB’s document-oriented approach means you can store data in a way that closely resembles the actual objects you use within your code, making it simple to understand and manipulate.
Advantages of Node.js Ecommerce:
Asynchronous and Non-blocking:
Node js is known for its asynchronous, event-driven architecture. In the context of e-commerce, this means handling a large number of simultaneous connections efficiently. For example, when multiple users are browsing products, Node js can handle these requests concurrently, ensuring a smooth and responsive user experience.
Single Language:
With Node js both server-side and client-side scripting can be done using JavaScript. This unification simplifies the development process, making it easier for developers to switch between the server and client sides seamlessly.
Vibrant Ecosystem:
Node js has a vast and active ecosystem of modules and packages available through npm (Node Package Manager). This allows developers to leverage existing solutions and easily integrate them into their e-commerce applications.
Scalability:
Node js is designed with scalability in mind. Its lightweight and event-driven architecture make it well-suited for building scalable applications that can handle a large number of concurrent connections.
MongoDB: A NoSQL Database
MongoDB is a popular NoSQL database that stores data in a flexible, JSON-like format called BSON. Its schema-less design allows for easy scalability and adaptability to changing data structures, making it an excellent choice for e-commerce applications with evolving requirements.
Advantages of MongoDB for E-commerce:
Flexible Schema:
MongoDB’s document-based data model provides flexibility in handling diverse data types. This is particularly beneficial in e-commerce, where product information, user data, and transaction details can vary significantly.
Horizontal Scalability:
MongoDB can scale horizontally by distributing data across multiple servers. This enables e-commerce applications to handle growing amounts of data and traffic by adding more servers to the database cluster.
Indexing and Query Performance:
MongoDB supports indexing, which can significantly improve query performance. This is crucial for e-commerce applications that need to retrieve and display product information quickly.
JSON-like Documents:
MongoDB stores data in BSON (Binary JSON) format, which is a JSON-like representation. This aligns well with the JSON data that is commonly used in web applications, making it easy to work with data across the entire stack.
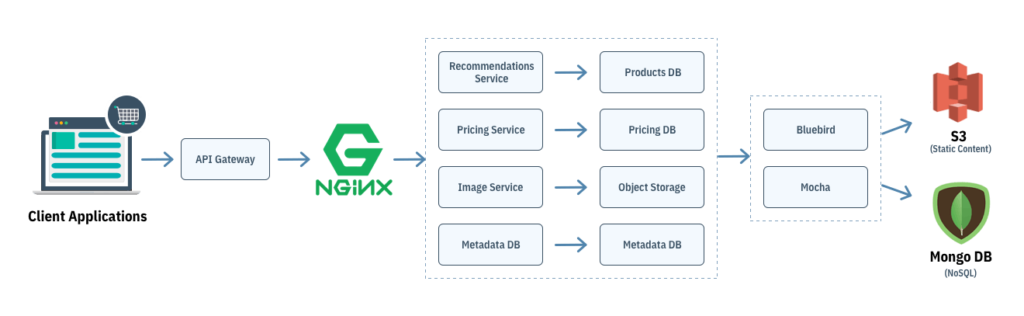
Building a Scalable Ecommerce node js Application
Example: Product Catalog
Let’s consider a simple example of building a scalable ecommerce node js product express and MongoDB.
1. Setting Up the Project:
mkdir scalable-ecommerce
cd scalable-ecommerce
npm init -y
npm install express mongoose
2. Creating the Express Server:
const express = require('express');
const app = express();
const PORT = 6363;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
3. Connecting to MongoDB:
const mongoose = require('mongoose');
mongoose.connect('mongodb://127.0.0.1:21017/ecommerce', { useNewUrlParser: true, useUnifiedTopology: true });
4. Defining the Product Model:
const productSchema = new mongoose.Schema({
name: String,
price: Number,
category: String,
});
const Product = mongoose.model('Product', productSchema);
5. Creating API Endpoints:
app.get('/products', async (req, res) => {
const products = await Product.find();
res.json(products);
});
app.post('/products', async (req, res) => {
const newProduct = req.body;
const product = await Product.create(newProduct);
res.json(product);
});
6. Scalability Considerations:
Implementing caching mechanisms (e.g., Redis) for frequently accessed data to reduce database load.
Load balancing to distribute incoming traffic across multiple servers.
Using a message queue (e.g., RabbitMQ) for handling asynchronous tasks, such as order processing.
Advantages and Disadvantages
Advantages:
Asynchronous and Non-blocking:
Node js utilizes an asynchronous, non-blocking I/O model, making it well-suited for handling concurrent operations. In e-commerce, where multiple users interact simultaneously, this ensures efficient handling of requests and improves the overall responsiveness of the application.
Example:
// Node js asynchronous code
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Real-time Capabilities:
Node js enables real-time communication through technologies like WebSockets, which is beneficial for features like live chat, notifications, and order tracking. Real-time updates enhance user engagement and provide a dynamic shopping experience.
Example:
// Setting up a WebSocket server with Node js
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 8080 });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
console.log(`Received: ${message}`);
});
ws.send('Hello, client!');
});
Scalability:
Node js and MongoDB are designed to scale horizontally, allowing for the addition of more resources to handle increased load. This is crucial for e-commerce platforms that experience varying levels of traffic, especially during promotions or seasonal sales.
Development Speed:
Node js facilitates rapid development due to its use of JavaScript on both the client and server sides. This can lead to faster time-to-market for e-commerce applications, which is advantageous in the competitive online marketplace.
Example:
// Simple Node js server
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, World!\n');
});
server.listen(6363, 'localhost', () => {
console.log('Server running at http://localhost:3000/');
});
Simple and Readable Code:
Node js combined with a framework like Express, allows developers to write clean and readable code. This simplicity accelerates the development process and makes the codebase maintainable.
Efficient Database Operations:
The asynchronous nature of Node js is evident in the database operations. Asynchronous calls to MongoDB ensure that the server is not blocked while waiting for database queries to complete, enhancing overall application performance.
Flexibility:
MongoDB’s flexible schema accommodates changes in data structure, crucial for adapting to the dynamic nature of e-commerce data.
Disadvantages:
Callback Hell:
The extensive use of callbacks in Node js can lead to callback hell, making code harder to read and maintain. While solutions like Promises and async/await can mitigate this issue, developers must be mindful of proper code structuring.
Not Ideal for CPU-Intensive Tasks:
While Node js excels in handling a large number of concurrent connections, it may not be the best choice for CPU-intensive tasks. Tasks that require significant computation may block the event loop, impacting overall application performance.
Maturity of the Ecosystem:
Node js ecosystem is vast, some modules might not be as mature or well-maintained as those in more established ecosystems, some enterprises may prefer more established stacks for mission-critical applications.
Complexity:
The flexibility offered by MongoDB’s schema-less design may introduce complexity in data management and querying.
Learning Curve:
Developers unfamiliar with JavaScript on the server-side or NoSQL databases may face a learning curve when adopting this technology stack.
Consistency and Transactions:
MongoDB, being a NoSQL database, sacrifices ACID transactions for improved scalability. In scenarios where data consistency is paramount, a relational database might be a more suitable choice.
A Mini Project for Aspiring Entrepreneurs
Dive into the world of e-commerce by taking on a mini project that will set you up with a solid foundation. This hands-on experience is invaluable for understanding how to create a platform that is not only responsive and user-friendly but also robust and scalable. With the combination of Node.js, Express and MongoDB you’ll have a stack that’s geared for success in the competitive e-commerce landscape.
Build Your Own Node.js E-Commerce Backend
Creating a Node.js e-commerce backend is a fantastic way to learn the ins and outs of e-commerce platforms. You’ll gain practical experience in handling product inventories, user authentication, payment processing, and much more. A strong backend is the engine of any e-commerce platform and mastering it can set you apart as a developer.
Conclusion
Building scalable e-commerce solutions requires a robust technology stack that can handle dynamic data, high traffic, and evolving requirements. Node js and MongoDB, with their strengths in scalability, performance, and flexibility, provide an excellent foundation for developing scalable e-commerce applications. By carefully considering the advantages and disadvantages, developers can harness the power of this stack to create resilient and efficient e-commerce platforms that can grow with the demands of the market.
FAQ
How does Node js contribute to scalable e-commerce solutions?
Node js with its non-blocking I/O and event-driven architecture empowers scalable e-commerce solutions. It efficiently handles concurrent requests, ensuring swift data processing and optimal performance for high-traffic e-commerce platforms.
advantages Of MongoDB In e-commerce?
MongoDB’s compatibility with Node js is pivotal for scalable e-commerce solutions. Its flexible schema and horizontal scalability complement Node js allowing for seamless data management and accommodating the growing needs of e-commerce platforms.
One Comment