Express API Rate Limit in Nodejs
In the dynamic world of Nodejs development managing web traffic effectively is crucial for optimal performance and security. One powerful tool in a developer’s collection is Express API rate limit, a feature designed to control and mitigate incoming requests to a server. This comprehensive guide explores the nuances of implementing and optimizing Express API rate limit in Nodejs applications.
What is Express API Rate Limit?
Express API rate limit is a middleware for the Express web application framework in Nodejs. It serves as a mechanism to control the rate at which clients can make requests to a server. By preventing abuse and potential security threats. Express rate limit contributes to a more stable and reliable web application.
The Importance of API Rate Limiting in Nodejs
In the Nodejs ecosystem, where asynchronous event-driven programming is the norm the need for efficient request handling is paramount. API Rate limiting ensures that a server is not overwhelmed by a sudden influx of requests preventing issues such as resource exhaustion and degraded performance.
How Express API Rate Limit Works in Nodejs
Express API rate limit operates by tracking the number of requests made by a client within a specified time frame. Once the predefined limit is reached subsequent requests from that client are either blocked or delayed. This process helps protect against common threats like Distributed Denial of Service (DDoS) attacks, ensuring a smoother experience for legitimate users.
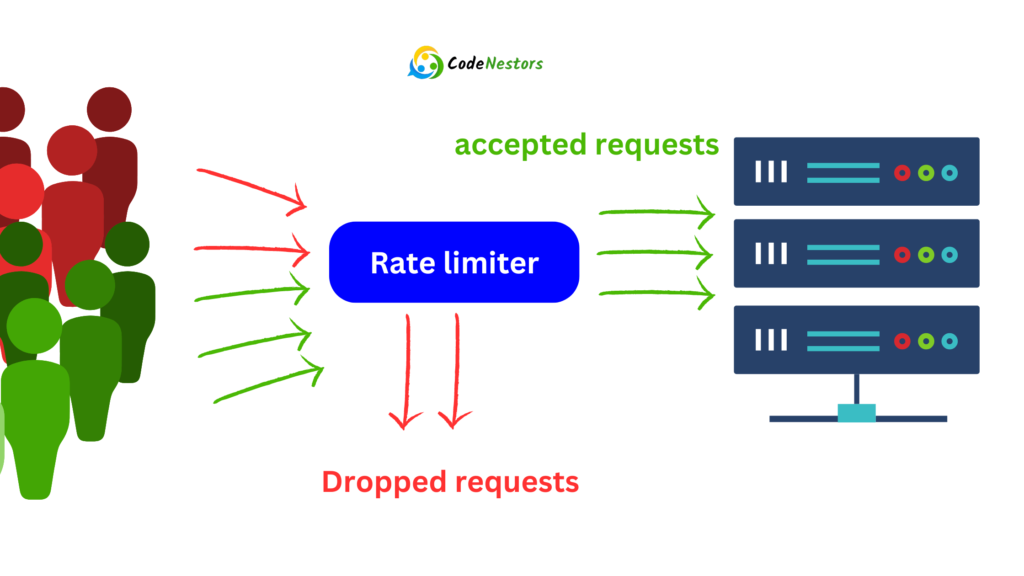
Implementing Express API Rate Limit in Nodejs
Adding rate limiting to your Nodejs application with Express is a straightforward process. First, install the express-rate-limit
middleware using npm:
npm install express-rate-limit
Now, integrate it into your Express application:
const express = require('express');
const rateLimit = require('express-rate-limit');
const app = express();
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
});
app.use(limiter);
This example sets up a rate limit of 100 requests per IP address in a 15-minute window. Adjust these values based on your application’s requirements.
Customizing Express API Rate Limit in Nodejs
Express API rate limit provides flexibility in customization. Developers can tailor rate limits based on specific routes, user roles or other criteria. For instance, you might want to impose stricter limits on certain API endpoints or relax restrictions for authenticated users.
const apiLimiter = rateLimit({
windowMs: 15 * 60 * 1000,
max: 50, // limit each IP to 50 requests per windowMs for APIs
});
// Apply to APIs
app.use('/api/', apiLimiter);
// Apply to other routes
app.use(limiter);
Building an API Rate Limiter in Nodejs with Express
Below is a simplified example of a mini-project for implementing API rate limiting in Nodejs using Express. This example uses the express-rate-limit
middleware.
Brief Of Nodejs API Rate limiter Project
- Install Dependencies:
- Ensure you have Nodejs and npm installed.
- Run
npm init -y
to initialize a new project. - Run
npm install express express-rate-limit
to install Express and the rate-limiting middleware.
- Create the Express App:
- Create a new file (e.g.,
app.js
) and paste the provided code.
- Create a new file (e.g.,
- Define Rate Limiting:
- Adjust the
windowMs
andmax
options in theapiLimiter
to set your desired rate limit. In this example, it’s set to 100 requests per IP every 15 minutes.
- Adjust the
- Create an API Endpoint:
- The
/api/data
endpoint is a placeholder for your actual API routes. You can extend the API with your logic.
- The
- Run the Application:
- Execute
node app.js
in the terminal to start the server.
- Execute
- Test the Rate Limit:
- Access the
/api/data
endpoint multiple times to observe the rate limiting in action.
- Access the
Overcoming Challenges with Express API Rate Limit in Nodejs
While Express rate limit is a powerful tool developers may encounter challenges such as false positives, where legitimate requests are blocked. To address this fine-tune the configuration based on your application’s traffic patterns and monitor the logs for any unexpected behavior.
Benefits of Using Express API Rate Limit in Nodejs
Implementing Express rate limit in your Nodejs application brings several benefits. It:
- Enhances Security: Mitigates the risk of abuse, including potential DDoS attacks.
- Improves Performance: Ensures fair resource allocation, preventing server overload.
- Optimizes User Experience: Maintains responsiveness for legitimate users by preventing abuse.
Conclusion
Express rate limit is a valuable asset for Nodejs developers, offering a robust solution to manage web traffic effectively. By implementing and customizing rate limits based on your application’s needs, you strike a balance between security and accessibility. Stay informed, adapt to emerging trends and empower your Nodejs applications with the resilience they need in the ever-evolving digital landscape.
FAQs
Can I use Express rate limit with other Nodejs frameworks?
Express rate limit is specifically designed for the Express framework, but similar rate-limiting solutions exist for other Nodejs frameworks.
Is Express rate limit suitable for all types of Nodejs applications?
Express rate limit is versatile and can be adapted for various applications. However, tailor the configuration based on the specific needs and usage patterns of your application.
Can Express rate limit be bypassed by malicious actors?
While rate limiting is effective against many threats no solution is entirely foolproof. Implement additional security measures and stay vigilant against evolving threats.