what is redux toolkit ? | what is use of redux toolkit ? | everything relevant about redux toolkit and pros in 2024
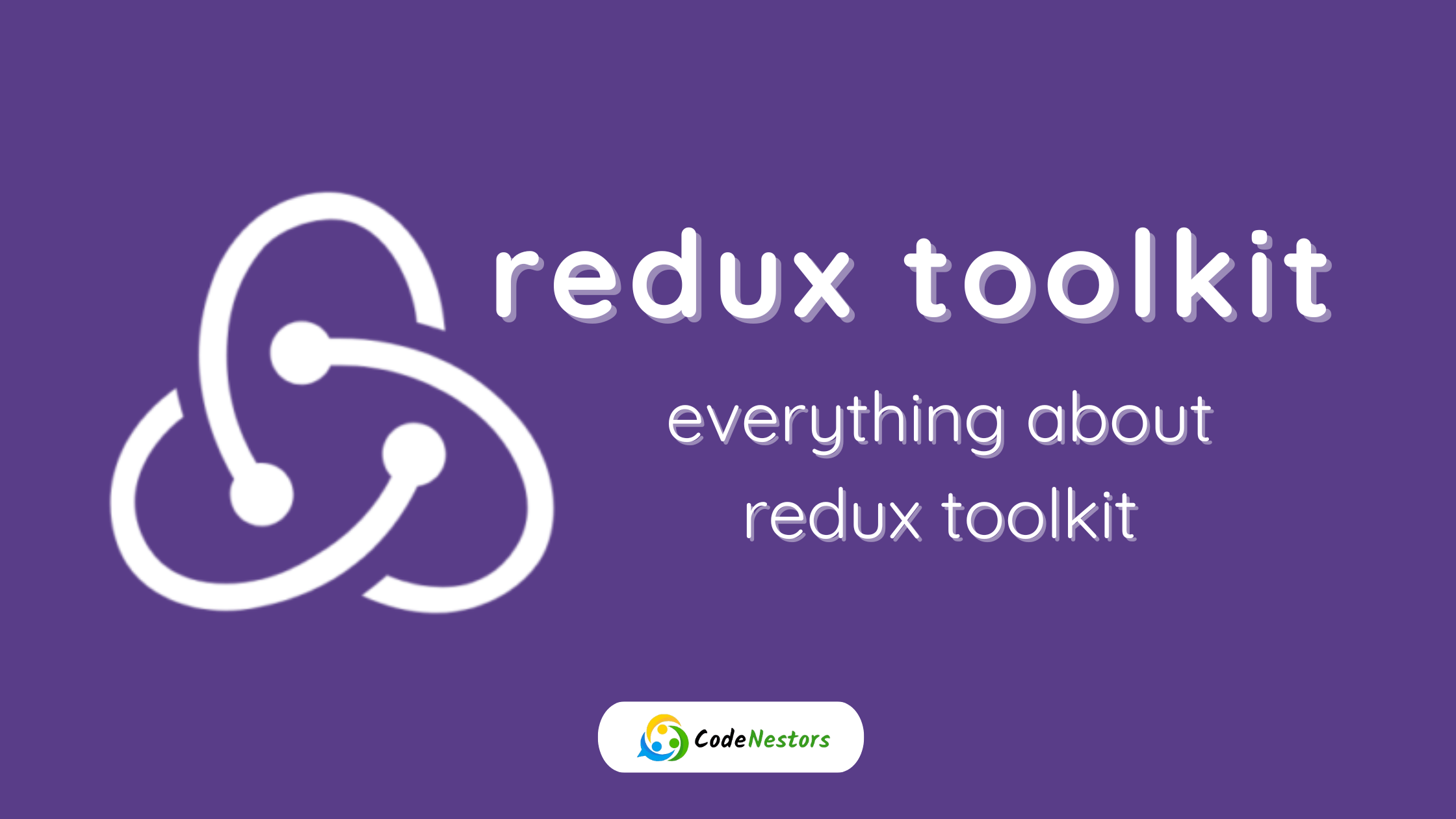
introduction
Building advanced and easy-to-maintain React apps requires effective state management. For a long time developers have relied on Redux but setting it up used to involve a bit of repetitive code. That’s where Redux Toolkit comes in. it’s like a handy toolbox from the Redux team to make things simpler. In this blog we will walk through Redux Toolkit checking out what makes it special & its main features & why it’s great finally how it makes handling state in React apps ease.
what is redux toolkit ?
Redux Toolkit is a set of utilities. officially recommended by the Redux team that simplifies and optimizes the usage of Redux in your applications. It is designed to address common challenges and streamline the development process making Redux more accessible and less verbose.
core features of redux toolkit
what is createSlice ?
createSlice is a utility function that reduces the amount of boilerplate code required to define Redux reducers and actions. It takes an initial state an object full of reducer functions and automatically generates action creators and a reducer.
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: state => state + 1,
decrement: state => state - 1,
},
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
what is configureStore ?
configureStore is a function that simplifies the setup of the Redux store. It automatically includes common middleware such as Redux DevTools and simplifies the overall store configuration process.
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './reducers';
const store = configureStore({
reducer: rootReducer,
});
what is createAsyncThunk ?
createAsyncThunk is a utility for handling asynchronous logic in Redux. It generates action creators that automatically dispatch pending fulfilled and rejected actions based on the promise resolution.
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit';
import api from './api';
export const fetchUserData = createAsyncThunk('user/fetchUserData', async userId => {
const response = await api.getUserData(userId);
return response.data;
});
const userSlice = createSlice({
name: 'user',
initialState: { data: {}, status: 'idle' },
reducers: {},
extraReducers: builder => {
builder
.addCase(fetchUserData.pending, state => {
state.status = 'loading';
})
.addCase(fetchUserData.fulfilled, (state, action) => {
state.status = 'succeeded';
state.data = action.payload;
})
.addCase(fetchUserData.rejected, state => {
state.status = 'failed';
});
},
});
export default userSlice.reducer;
Immutability Helpers: Updating State Immutably
Redux Toolkit utilizes immer under the hood allowing you to write code that looks like it’s mutating the state directly. immer ensures that the state is updated immutably. providing a clear and efficient way to manage state changes.
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: state => {
state.value += 1;
},
decrement: state => {
state.value -= 1;
},
},
});
Redux DevTools Integration
Redux Toolkit seamlessly integrates with Redux DevTools. providing a powerful set of debugging tools. It enhances your ability to inspect the state ,review actions and understand the flow of your application’s state changes.
import { configureStore } from '@reduxjs/toolkit';
const store = configureStore({
reducer: rootReducer,
devTools: process.env.NODE_ENV !== 'production', // Enable DevTools only in development
});
what is createEntityAdapter ?
Redux Toolkit includes an createEntityAdapter utility that simplifies the normalization of entity state. This is particularly useful when dealing with data structures where entities have unique IDs such as managing a collection of items fetched from an API.
import { createSlice, createEntityAdapter } from '@reduxjs/toolkit';
const usersAdapter = createEntityAdapter();
const usersSlice = createSlice({
name: 'users',
initialState: usersAdapter.getInitialState(),
reducers: {
addUser: usersAdapter.addOne,
updateUser: usersAdapter.updateOne,
removeUser: usersAdapter.removeOne,
},
});
export const { addUser, updateUser, removeUser } = usersSlice.actions;
export const { selectById: selectUserById, selectAll: selectAllUsers } = usersAdapter.getSelectors(state => state.users);
export default usersSlice.reducer;
Middleware Simplification
Redux Toolkit simplifies the inclusion of middleware making it easier to integrate third-party middleware or custom middleware into your Redux store.
import { configureStore, getDefaultMiddleware } from '@reduxjs/toolkit';
import loggerMiddleware from 'redux-logger';
const middleware = [...getDefaultMiddleware(), loggerMiddleware];
const store = configureStore({
reducer: rootReducer,
middleware,
});
Benefits of Using Redux Toolkit
Reduced Boilerplate Code
One of the primary benefits of Redux Toolkit is the significant reduction in boilerplate code. The concise syntax and simplified API allow developers to focus more on application logic and less on the setup and configuration of Redux.
Simplified Async Logic Handling
Handling asynchronous logic in Redux becomes more straightforward with the inclusion of createAsyncThunk. This utility simplifies the process of managing API calls and other asynchronous operations. improving the overall developer experience.
Ergonomic API
Redux Toolkit provides an ergonomic API that is designed to be more developer-friendly. The utilities and conventions it introduces contribute to a more enjoyable and efficient development process.
Improved Performance with Immutability Helpers
By utilizing immer for immutability. Redux Toolkit ensures that state updates are performed with optimal performance and minimal overhead. This results in a smoother and more responsive application.
Advanced Features and Best Practices for redux toolkit
Redux DevTools Configuration
While Redux DevTools are integrated seamlessly. you can further configure them to suit your needs. Customize the settings. use time-travel debugging and gain insights into the evolution of your application’s state.
import { configureStore } from '@reduxjs/toolkit';
const store = configureStore({
reducer: rootReducer,
devTools: {
name: 'MyApp',
features: {
jump: false,
},
},
});
Selectors with createSlice
Redux Toolkit allows you to define selectors within the createSlice function making it convenient to access and derive values from the state.
const userSlice = createSlice({
name: 'user',
initialState: { data: {}, status: 'idle' },
reducers: {},
extraReducers: builder => {
// ...reducer logic
},
selectors: {
selectUserData: state => state.data,
selectUserStatus: state => state.status,
},
});
export const { selectUserData, selectUserStatus } = userSlice.selectors;
export default userSlice.reducer;
CombineReducers Simplification
Redux Toolkit simplifies the process of combining reducers with the combineReducers utility. reducing the boilerplate associated with managing multiple slices of state.
import { combineReducers } from '@reduxjs/toolkit';
import userReducer from './userSlice';
import postsReducer from './postsSlice';
const rootReducer = combineReducers({
user: userReducer,
posts: postsReducer,
});
Thunks and Async Logic
Redux Toolkit enhances the handling of asynchronous logic by encapsulating async logic within thunks. Thunks are functions that can be dispatched and can contain asynchronous logic before dispatching further actions.
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit';
import api from './api';
export const fetchUserData = createAsyncThunk('user/fetchUserData', async userId => {
const response = await api.getUserData(userId);
return response.data;
});
const userSlice = createSlice({
name: 'user',
initialState: { data: {}, status: 'idle' },
reducers: {},
extraReducers: builder => {
builder
.addCase(fetchUserData.pending, state => {
state.status = 'loading';
})
.addCase(fetchUserData.fulfilled, (state, action) => {
state.status = 'succeeded';
state.data = action.payload;
})
.addCase(fetchUserData.rejected, state => {
state.status = 'failed';
});
},
});
export default userSlice.reducer;
Best Practices for Using Redux Toolkit
Use createSlice for Reducers
When defining reducers prefer using the createSlice function. It encapsulates the reducer logic, action types and action creators within a single module and reducing the need for additional boilerplate.
Leverage Immer for Immutability
Take advantage of the immutability helpers provided by immer when updating state within reducers. This allows you to write code that appears to mutate state directly while ensuring that the updates are performed immutably.
Structured Folder Organization
Organize your Redux-related files in a structured manner. Group reducers, actions and selectors by feature or domain to maintain a clean and maintainable project structure.
CombineReducers for Scalability
As your application grows use combineReducers to manage multiple slices of state. This allows you to break down your application’s state into smaller and manageable pieces and reduces the complexity associated with a monolithic store.
concusion
Redux Toolkit is a game-changer in the world of state management with Redux. Its collection of utilities significantly reduces boilerplate code, simplifies the setup process and enhances the overall developer experience. By adopting Redux Toolkit developers can focus more on building features and less on managing the intricacies of state in their applications.
This comprehensive guide covered the core features of Redux Toolkit, its benefits and best practices for usage. Armed with this knowledge. you are well-equipped to harness the power of Redux Toolkit in your React applications. As you dive deeper into Redux Toolkit explore its advanced features and implement best practices. you will discover how it transforms the way you approach state management in React.
hope this is helpfull !
What is Redux Toolkit, and why should I use it?
Redux Toolkit is an official set of tools designed to simplify and enhance the development experience with Redux. It reduces boilerplate code, streamlines state management and provides utilities to handle common Redux use cases. Use it to make your Redux development more efficient and enjoyable.
How does Redux Toolkit differ from traditional Redux?
Redux Toolkit streamlines Redux development by providing utilities like createSlice for defining reducers and configureStore for easy store setup and createAsyncThunk for handling asynchronous logic. It reduces the amount of boilerplate code traditionally associated with Redux.
What is createSlice, and why is it useful?
createSlice is a utility that combines reducer logic with action creators. It helps you define slices of your Redux state in a more concise way. With createSlice you write less boilerplate and get action creators and a reducer in one package.
How does Redux Toolkit enhance immutability?
Redux Toolkit utilizes immer under the hood allowing you to write code that looks like it’s mutating the state directly. immer ensures that state updates are performed immutably enhancing performance and readability.
Can I use Redux Toolkit for small projects?
Absolutely, While Redux Toolkit is excellent for large-scale applications. its simplicity and reduced boilerplate make it a great choice for small projects as well. It adapts to the needs of both simple and complex applications.