Handling cross-origin using Next js CORS
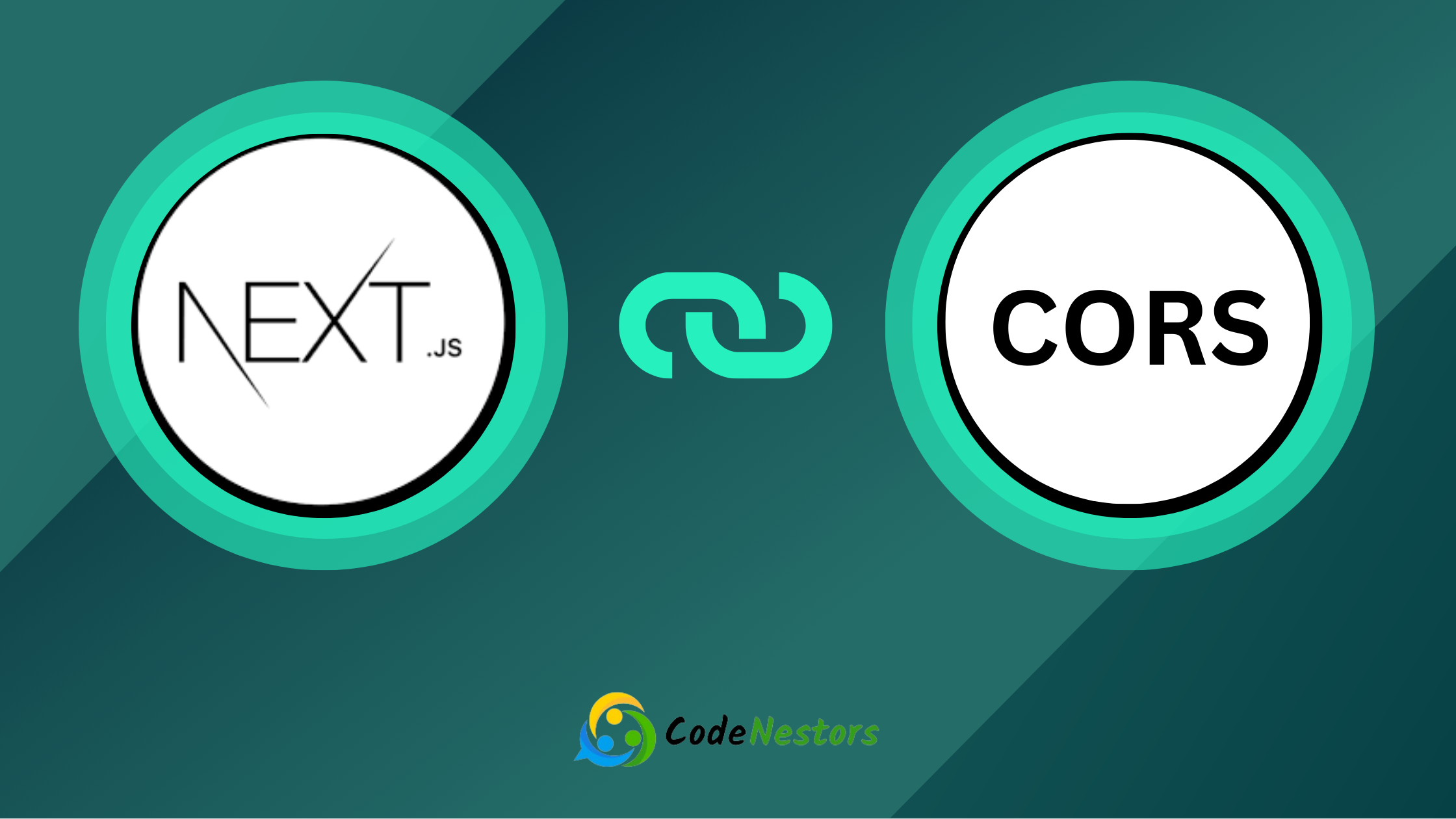
In recent years, Next.js has solidified its position as a primary framework, not only catering to frontend development but also emerging as a compelling choice for building resilient APIs. As developers increasingly turn to Next.js for its versatility, the importance of addressing Cross-Origin Resource Sharing (CORS) in the context of Next js CORS becomes paramount. This article delves into the dual role of Next.js, emphasizing its significance in frontend and API development, while specifically examining the critical considerations surrounding CORS within the Next.js ecosystem. Understanding and implementing effective CORS strategies in Next.js is essential for ensuring secure and seamless communication between web applications, ultimately contributing to a robust and interoperable development environment.
What is CORS?
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control the access that a web page from one domain has to resources on another domain. In essence, it is a set of rules that govern how web pages hosted on one domain can request and interact with resources, such as data or services, from a different domain.
Due to the same-origin policy followed by browsers, web pages are typically only allowed to make requests to the same domain from which they originated. CORS relaxes this policy, enabling web applications to make cross-origin requests under controlled circumstances. When a web page hosted on one domain makes a cross-origin request to another domain, the server on the receiving end can include specific CORS headers in its response. These headers convey permissions to the browser, allowing or restricting the requested resource access based on the rules defined by the server.
CORS is crucial for web security, preventing malicious websites from making unauthorized requests to a different domain on behalf of a user. It provides a mechanism for servers to declare which origins are permitted to access their resources, thereby maintaining a balance between security and the need for cross-origin communication in web development.
Types of CORS headers
- Access-Control-Allow-Origin:
This header indicates which origins are permitted to access the resource. It can be a specific origin, a comma-separated list of origins, or a wildcard (*) indicating that any origin is allowed.
Example:
Access-Control-Allow-Origin: https://example.com
- Access-Control-Allow-Methods:
Specifies the HTTP methods (e.g., GET, POST, PUT) that are allowed when making the actual request to the resource.
Example:
Access-Control-Allow-Methods: GET, POST, PUT
- Access-Control-Allow-Headers:
Indicates which HTTP headers can be used when making the actual request. It lists the allowed headers.
Example:
Access-Control-Allow-Headers: Content-Type, Authorization
- Access-Control-Allow-Credentials:
This header is used to indicate whether the browser should include credentials (such as cookies or HTTP authentication) when making the actual request.
Example:
Access-Control-Allow-Credentials: true
- Access-Control-Expose-Headers:
Lists the headers that the browser should expose to the requesting client when making the actual request.
Example:
Access-Control-Expose-Headers: X-Custom-Header
- Access-Control-Max-Age:
Specifies the maximum time (in seconds) that the results of a preflight request (a preliminary request sent before the actual request) can be cached.
Example:
Access-Control-Max-Age: 86400
Why Next js CORS Matters
Enhancing Security and Accessibility
Next js CORS acts as a gatekeeper, regulating which external domains can access your resources. This not only fortifies your website’s security but also enhances accessibility, ensuring a smooth interaction for users across various platforms.
Streamlining API Requests
Efficient API communication is at the heart of web applications. With Next js CORS, you can streamline API requests, minimizing latency and optimizing the overall performance of your website. This translates to a faster and more responsive user experience.
Setting Up Next.js with CORS
Step 1: Install Next.js
Make sure you have Node.js installed on your system. If not, you can download it from nodejs.org.
Open your terminal and run the following command to install Next.js globally:
npm install -g create-next-app
Step 2: Create a Next.js Project
Create a new Next.js project using the following commands:
npx create-next-app my-nextjs-project
cd my-nextjs-project
Step 3: Install CORS Middleware
Now, install the cors
middleware using the following command:
npm install cors
Step 4: Configure CORS in Next.js (server side)
Create a custom server using the express
framework to configure CORS. In your project root, create a file named server.js
with the following content:
// server.js
const express = require('express');
const next = require('next');
const cors = require('cors');
const dev = process.env.NODE_ENV !== 'production';
const app = next({ dev });
const handle = app.getRequestHandler();
app.prepare().then(() => {
const server = express();
// Enable CORS
server.use(cors());
server.all('*', (req, res) => {
return handle(req, res);
});
server.listen(3000, (err) => {
if (err) throw err;
console.log('> Ready on http://localhost:3000');
});
});
Step 5: Update package.json
In your package.json
file, add the following scripts to start the server with the custom configuration:
{
"scripts": {
"dev": "node server.js",
"build": "next build",
"start": "NODE_ENV=production node server.js"
}
}
Step 6: Client-Side Configuration:
If your client is making requests to the server, ensure that the requests include the necessary headers. For example, in your client-side code, you might use the fetch
API:
fetch('http://localhost:3000/api/data', {
method: 'GET',
headers: {
'Content-Type': 'application/json',
// Add any other headers as needed for your application
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace the URL and adjust headers according to your needs.
Step 7: Test CORS Setup
Start your Next.js project using the custom server:
npm run dev
Visit http://localhost:3000
in your browser and test if CORS is properly configured.
Remember to replace http://localhost:3000
with the actual URL where your Next.js application is hosted in a production environment.
Client-Side vs. Server-Side CORS in Next.js
- Client-Side CORS:
- Definition: Client-side CORS refers to handling CORS on the browser or client side. It involves making requests from a web application running in a browser to a server on a different domain.
- Implementation in Next.js: When dealing with client-side CORS in Next.js, you primarily focus on configuring your client-side code to send proper CORS headers and handle responses. This is commonly done using the
fetch
API or third-party libraries like Axios. - Example: In a Next.js project, if you are using the
fetch
API to make requests to an external API, you may need to ensure that the server hosting the API allows requests from your Next.js application’s domain. This involves setting up proper headers on the API server to allow cross-origin requests.
- Server-Side CORS:
- Definition: Server-side CORS involves configuring the server to include appropriate headers in its responses, indicating which origins are permitted to access its resources. This is crucial when your Next.js server serves APIs or resources that may be requested by client applications running on different domains.
- Implementation in Next.js: In a Next.js project, server-side CORS can be handled by configuring the server to include the necessary CORS headers. This is often done using middleware or server settings, depending on the server technology you are using with Next.js (e.g., Express, Koa).
- Example: If your Next.js application includes an Express server, you might use the
cors
middleware to handle CORS. This middleware can be configured to allow or restrict requests based on the origin of the incoming requests.
FAQs
Is CORS necessary in every Next.js project?
While not mandatory, implementing CORS in Next.js projects is advisable, especially when dealing with cross-origin requests.
What is Cross-Origin Resource Sharing (CORS) and why is it important in web development?
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control access to resources on a web page from a different domain. It is crucial for maintaining security while allowing legitimate cross-origin requests.
How does Next.js handle CORS by default?
Next.js handles CORS by default, allowing same-origin requests. This means that requests made from the same domain are automatically permitted, while cross-origin requests are blocked.
Can I customize CORS settings in Next.js?
Yes, you can customize CORS settings in Next.js. You can use the next.config.js
file to define custom headers, including CORS headers, using the headers
property.
How can I enable CORS for specific routes in Next.js?
To enable CORS for specific routes, you can use the headers
property within the getServerSideProps
or getInitialProps
functions for those specific pages. Set the Access-Control-Allow-Origin
header accordingly.
Can I disable CORS in development mode for easier testing?
Yes, in development mode, you can disable CORS by setting the CORS_ORIGIN
environment variable to true
. However, this should be used cautiously and only for testing purposes.