Why We Should Use Session, Cookies and Middleware in Nodejs
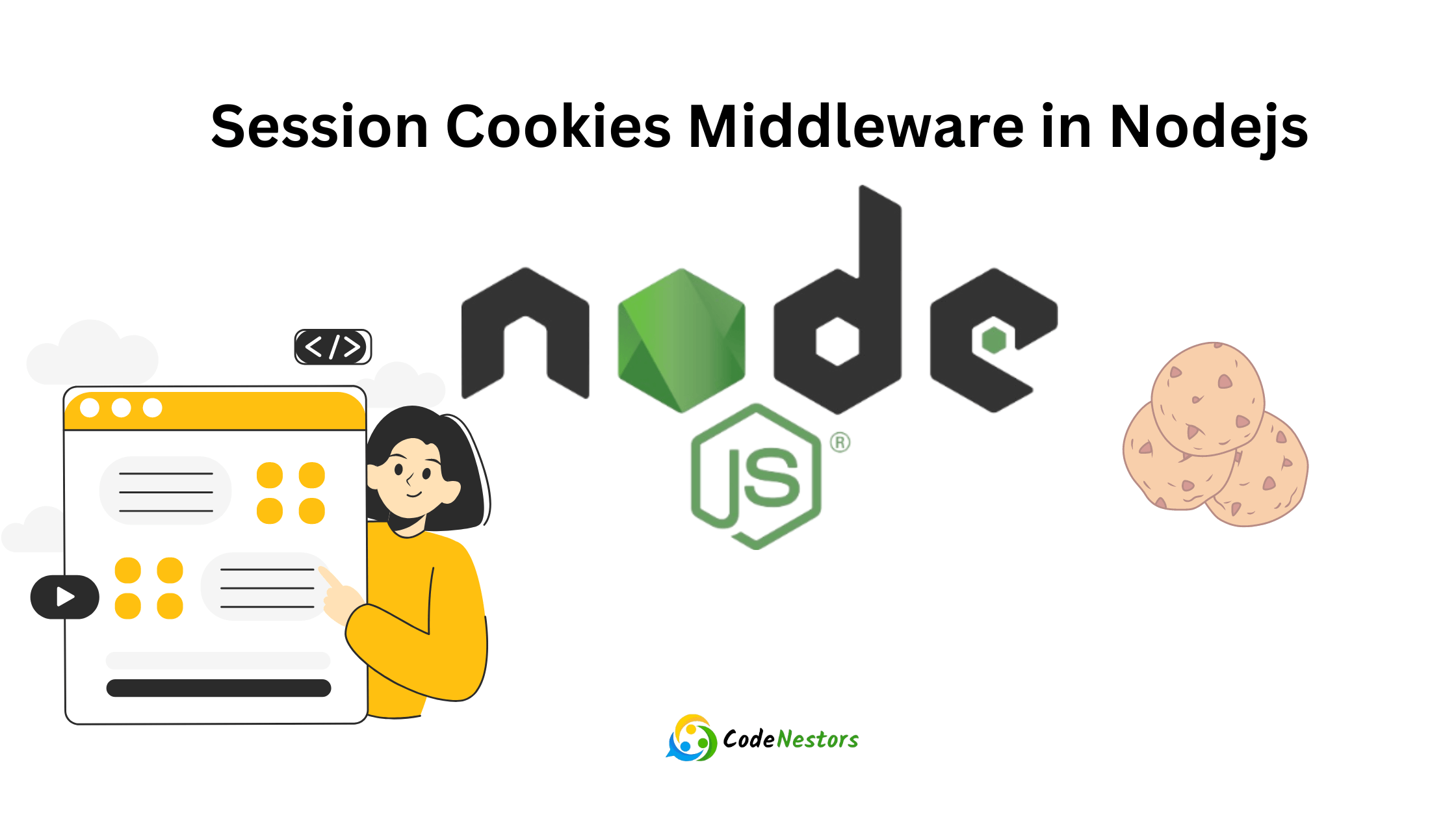
In the realm of web development, managing user session and state is pivotal for delivering personalized, secure and dynamic experiences. Node.js empowers developers with tools like session, cookies and middleware to skillfully handle this aspect. This guide aims to unravel the intricacies of these mechanisms, their implementation, prerequisites and their crucial role in crafting robust web applications.
Managing user session and state in web applications is crucial for maintaining user authentication, personalization and interactions. In Node.js, developers leverage session, cookies and middleware to handle this aspect efficiently. In this guide, we’ll delve deep into these mechanisms, their implementation, advantages and drawbacks.
Understanding Session in Nodejs: The Inner Workings
What are Session
Session embody the ongoing interaction between users and web applications, preserving user-specific data during their visit. They rely on unique identifiers to track and manage user information. Session represent the stateful interaction between a user and a web application over a specific period. They allow the server to store user-specific data during the user’s visit.
How Session Work
- Session Initialization: Upon user interaction, a session begins, assigning a unique session ID.
- Data Storage: Session data, stored on the server or in a database, corresponds to the session ID.
- Session Handling: As users navigate the application, session data is accessed and modified as needed.
- Session Termination: Session conclude either upon user logout or after a period of inactivity.
Prerequisites for Session Handling
- Express.js: Node.js developers primarily leverage the express-session package for streamlined session management.
- Middleware Knowledge: Understanding middleware in Express is beneficial for effectively integrating session management into the application’s flow.
Implementing Session
In Node.js, the express-session package is commonly used to handle session. Here’s an example:
const express = require('express');
const session = require('express-session');
const app = express();
app.use(session({
secret: 'yourSecretKey',
resave: false,
saveUninitialized: true
}));
// Using sessions in routes
app.get('/', (req, res) => {
// Access session data
if (req.session.views) {
req.session.views++;
} else {
req.session.views = 1;
}
res.send(`Views: ${req.session.views}`);
});
app.listen(3000);
Advantages of Session
- Granular Control: Session allow the storage of diverse user-related information, enabling tailored experiences and personalized content delivery.
- Authentication Management: Ideal for maintaining user authentication status throughout their browsing session.
- E-commerce Applications: Suited for retaining shopping cart items and user preferences during an online shopping session.
- Server-Side Storage: Session data is stored on the server, making it more secure.
- Flexibility: Allows storing various types of data associated with a user’s session.
Disadvantages of Session
- Server Load: Storing session data on the server can increase server load, especially for large applications.
- Scaling Challenges: Scaling across multiple servers can be complex due to maintaining session data consistency.
Exploring Cookies in Nodejs: Tracking User Preferences
What are Cookies?
Cookies, small data pieces stored in a user’s browser, serve various purposes, including retaining user preferences, session management and tracking user behavior.
How Cookies Work
- Cookie Creation: The server sends a response containing a Set-Cookie header to the client, storing the cookie in the browser.
- Data Storage: Cookies store key-value pairs, retaining information such as user preferences or session identifiers.
- Client-Server Communication: Subsequent requests from the client include the stored cookie data in the Cookie header.
- Expiration and Security: Cookies can have expiration dates and security flags for encryption.
Prerequisites for Cookie Handling
- Express.js with Cookie Middleware: Utilizing cookie-parser in conjunction with Express simplifies cookie management and parsing.
- Understanding HTTP Protocol: Knowledge of HTTP headers and how cookies are exchanged between the client and server is beneficial.
Implementing Cookies
Node.js provides the cookie-parser middleware to handle cookies. Example:
const express = require('express');
const cookieParser = require('cookie-parser');
const app = express();
app.use(cookieParser());
app.get('/', (req, res) => {
let visits = parseInt(req.cookies.visits) || 0;
visits++;
res.cookie('visits', visits, { maxAge: 86400000 }); // Set cookie expiration (1 day)
res.send(`Visits: ${visits}`);
});
app.listen(3000);
Advantages of Cookies
- Client-Side Storage: Perfect for storing data on the client’s side, minimizing server loads and enhancing performance.
- Personalization: Ideal for remembering user preferences and settings across multiple visits to a website.
- Tracking User Behavior: Valuable for analytics and targeted advertising purposes.
- Client-Side Storage: Data is stored on the client-side, reducing server load.
- Simplicity: Easy to implement and use for basic session management.
Disadvantages of Cookies
- Security Vulnerabilities: Prone to security risks like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF).
- Limited Storage Capacity: Imposes constraints on the amount of data that can be stored in cookies.
- Security Concerns: Vulnerable to attacks like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF).
- Limited Storage: Size limitations exist for cookie data storage.
Embracing Middleware in Nodejs: Sculpting the Application Flow
What is Middleware
Middleware functions in Node.js allow you to perform tasks before sending a response to the client. They can handle session, manipulate request and response objects, enabling tasks such as authentication, logging and session handling.
How Middleware Works
- Request Interception: Middleware functions intercept incoming requests before reaching the intended route handler.
- Task Execution: Middleware can execute tasks like authentication checks, logging, or data preprocessing.
- Response Manipulation: They can modify the response before sending it back to the client.
Prerequisites for Middleware Usage
- Express.js Application Setup: Middleware functions are integrated into Express using app.use() before route handlers.
- JavaScript and Node.js Fundamentals: Proficiency in JavaScript and Node.js basics facilitates custom middleware creation.
Implementing Middleware
Example of a simple middleware logging requests:
const express = require('express');
const app = express();
app.use((req, res, next) => {
console.log(`Request received: ${req.method} ${req.url}`);
next();
});
// Other route handlers...
app.get('/', (req, res) => {
res.send('Hello, middleware!');
});
app.listen(3000);
Advantages of Middleware
- Modularity and Extensibility: Facilitates the segregation of concerns and seamless integration of additional functionalities.
- Request-Response Manipulation: Enables authentication checks, error handling,and request preprocessing.
- Modularization: Allows separating concerns and organizing code effectively.
- Extensibility: Easily integrate additional functionalities into the request-response cycle.
Disadvantages of Middleware
- Ordering Complexity: The order of middleware execution can significantly impact application behavior, leading to subtle bugs.
- Performance Overhead: Excessive use of middleware might affect performance due to increased processing time.
- Ordering Complexity: The order of middleware execution can impact the application’s behavior, leading to subtle bugs.
- Overhead: Using numerous middleware can affect performance.
Why Session and State Management is Essential in Web Development
- Enhanced User Experience: Seamlessly managing session and states ensures smoother user interactions, resulting in higher user satisfaction.
- Security Assurance: Proper session management mitigates security risks, safeguarding user data and application integrity.
- Personalization and Customization: Effective state management allows for tailored experiences, catering to individual user preferences
Conclusion
Efficient management of session, cookies and middleware in Node.js empowers developers to create robust, responsive and secure web applications. Each mechanism offers unique advantages and considerations, requiring careful evaluation based on project requisites.
Embracing session for secure server-side storage, utilizing cookies for client-side simplicity and leveraging middleware for granular control offers a versatile toolkit. Choosing the right strategy hinges on specific application contexts and objectives.
FAQs
Can sessions and cookies complement each other in Node.js?
Yes, sessions often utilize cookies to store session identifiers while preserving data on servers.
Are cookies suitable for storing sensitive data securely?
While encryption and secure flags enhance security, cookies are susceptible to certain vulnerabilities.
Is storing extensive data in sessions or cookies advisable?
Large data storage in sessions or cookies isn’t recommended due to limitations and potential performance impact.
Which state management approach aligns best with microservices architecture?
Stateless approaches like JWTs (JSON Web Tokens) are often favored in microservices for scalability and simplicity.
Understanding these mechanisms empowers developers to make informed decisions, orchestrating efficient session and state management strategies, elevating their Node.js applications to new levels of performance, security, and user satisfaction.
One Comment