Deep Dive into Error Handling and Logging in Node js
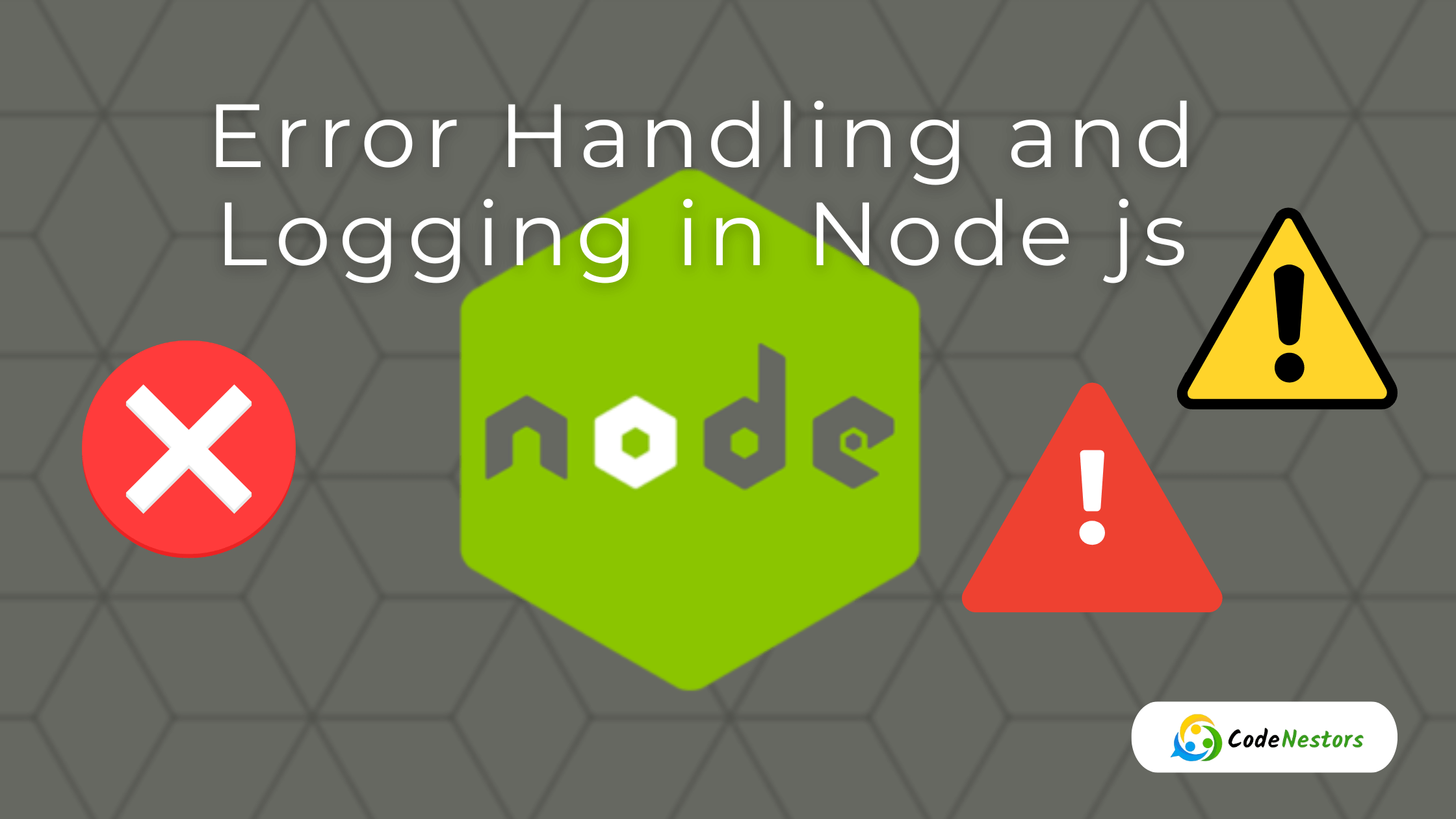
In the realm of Node.js backend development, the meticulous handling of errors and comprehensive logging are indispensable for maintaining application stability, streamlining debugging, and ensuring a smooth user experience. This in-depth guide dissects their significance, advantages, disadvantages, and offers a hands-on exploration of best practices.
Advantages of Robust Error Handling and Logging in Node js
Streamlined Debugging Process :
Efficient error handling coupled with detailed logs significantly expedites the debugging process. It allows developers to swiftly trace and rectify issues, ultimately reducing downtime and enhancing the user experience. It also help in reducing debugging time and enhancing troubleshooting capabilities.
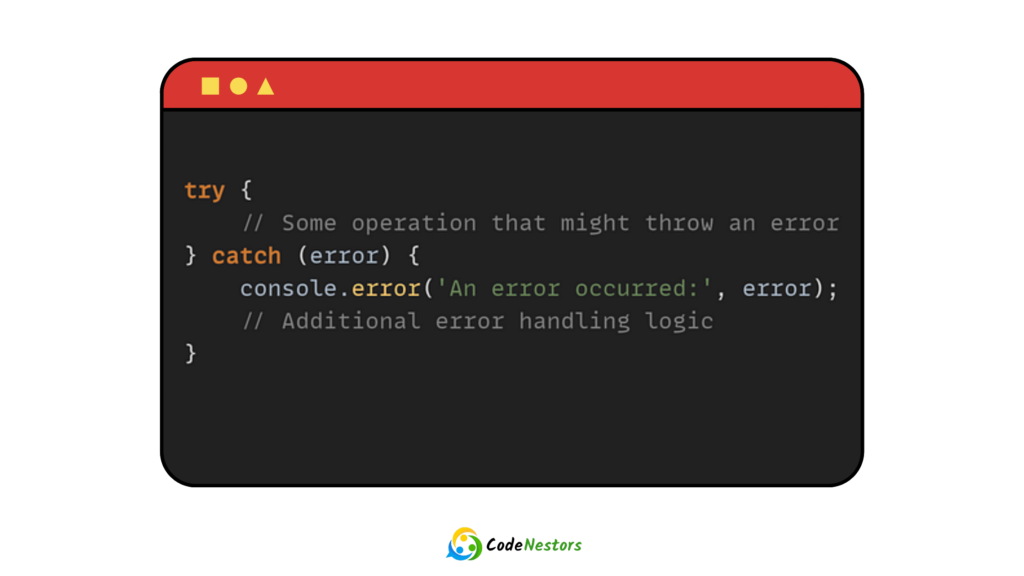
Enhanced Application Stability and Reliability :
Strategically handled errors act as safety nets, preventing unexpected crashes or disruptions. They instill confidence in the application’s stability, thereby fortifying its reliability. Properly handled errors and comprehensive logs contribute to increased application stability, allowing developers to catch and resolve issues before they escalate.
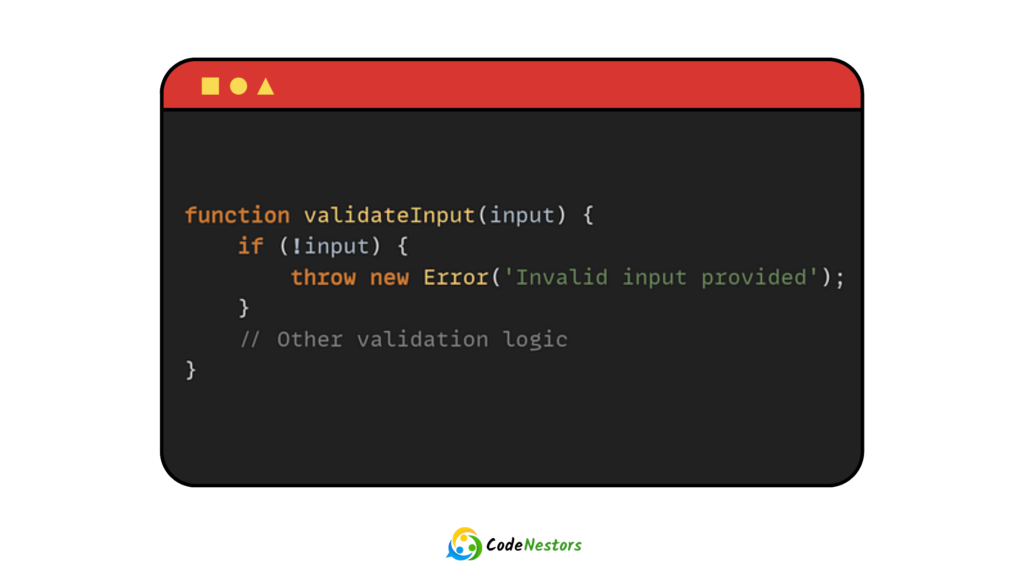
Insights for Performance Optimization :
Comprehensive logs serve as treasure troves of insights into system behavior. They play a pivotal role in identifying bottlenecks, inefficiencies, and aiding in performance optimization.
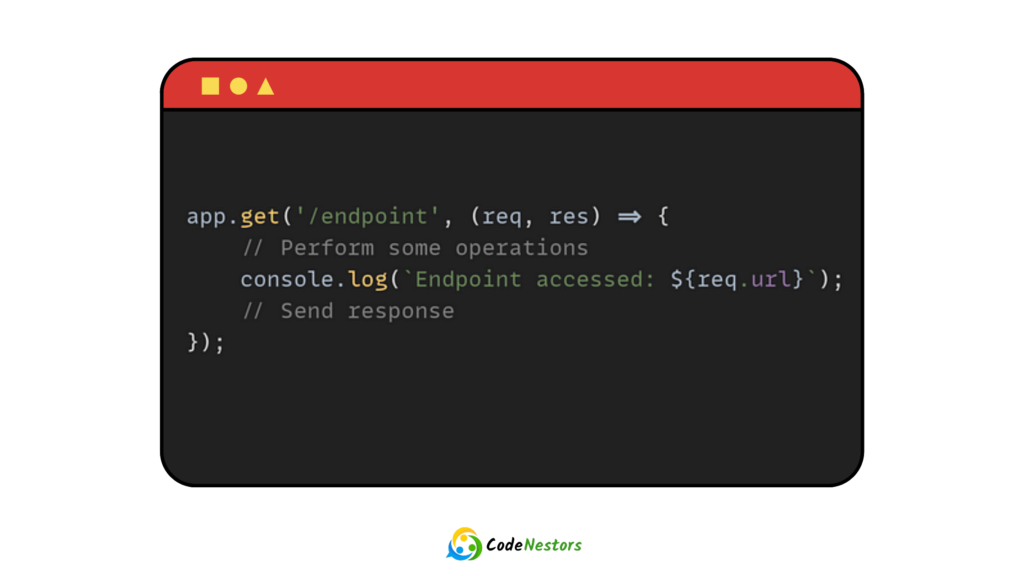
Disadvantages of Inadequate Error Handling and Logging
Reduced Diagnosing Efficiency :
Insufficient error messages or logs significantly impede developers ability to diagnose issues efficiently. Vague error descriptions prolong debugging cycles and hinder quick resolutions.
Difficulty in Root Cause Analysis :
Lack of detailed error messages or logs makes root cause analysis challenging to diagnose and resolve problems effectively. It becomes arduous to identify the core issues, leading to delays in problem resolution.
Best Practices for Error Handling and Logging
Consistent Error Handling Patterns :
Consistency in error handling is paramount. Whether utilizing try-catch blocks, Promises, or async/await, maintaining uniformity across the codebase fosters clarity and ease of maintenance.
Specialized Error Classes :
Adopting specialized error classes facilitates a granular approach to error classification and handling. It enables developers to discern between different types of errors, enhancing the troubleshooting process.
Implement Robust Logging Libraries :
Robust logging libraries such as Winston or Bunyan provide powerful tools for generating detailed logs with varying levels of severity and pertinent contextual information.
Contextual Information in Logs :
Incorporating contextual information, such as request IDs, user details, or system configurations, within logs enriches their value. It simplifies debugging and accelerates issue resolution.
Centralized Log Monitoring :
Centralized log monitoring tools empower developers by aggregating and analyzing logs, facilitating proactive identification of anomalies or patterns, and expediting issue resolution.
Example of Error Handling
function fetchData() {
return new Promise((resolve, reject) => {
// Simulated database query
const data = null; // after get from perameteres
if (!data) {
// Error not properly handled
reject('Data not found');
}
resolve(data);
});
}
// Incorrect: Calling fetchData without error handling //
fetchData()
.then((data) => {
console.log(data);
});
// Correct: Implementing error handling with catch
fetchData()
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
Example of Logging Strategies
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
function processOrder(order) {
try {
// Process order logic
if (order.items.length === 0) {
throw new Error('No items in order');
}
// ...
logger.info('Order processed successfully');
} catch (error) {
logger.error('Error processing order:', error);
}
}
Conclusion
In the dynamic landscape of Node.js backend development, error handling and logging emerge as fundamental pillars. While adept error handling and logging offer advantages like accelerated debugging and enhanced stability, inadequate practices can impede issue diagnosis and root cause analysis. By meticulously implementing consistent error handling, leveraging specialized error classes, adopting robust logging strategies, and harnessing centralized monitoring, developers can fortify their applications, streamline debugging, and ensure a more resilient backend infrastructure.
One Comment