Handling File Uploads Using Multer in Node js Express
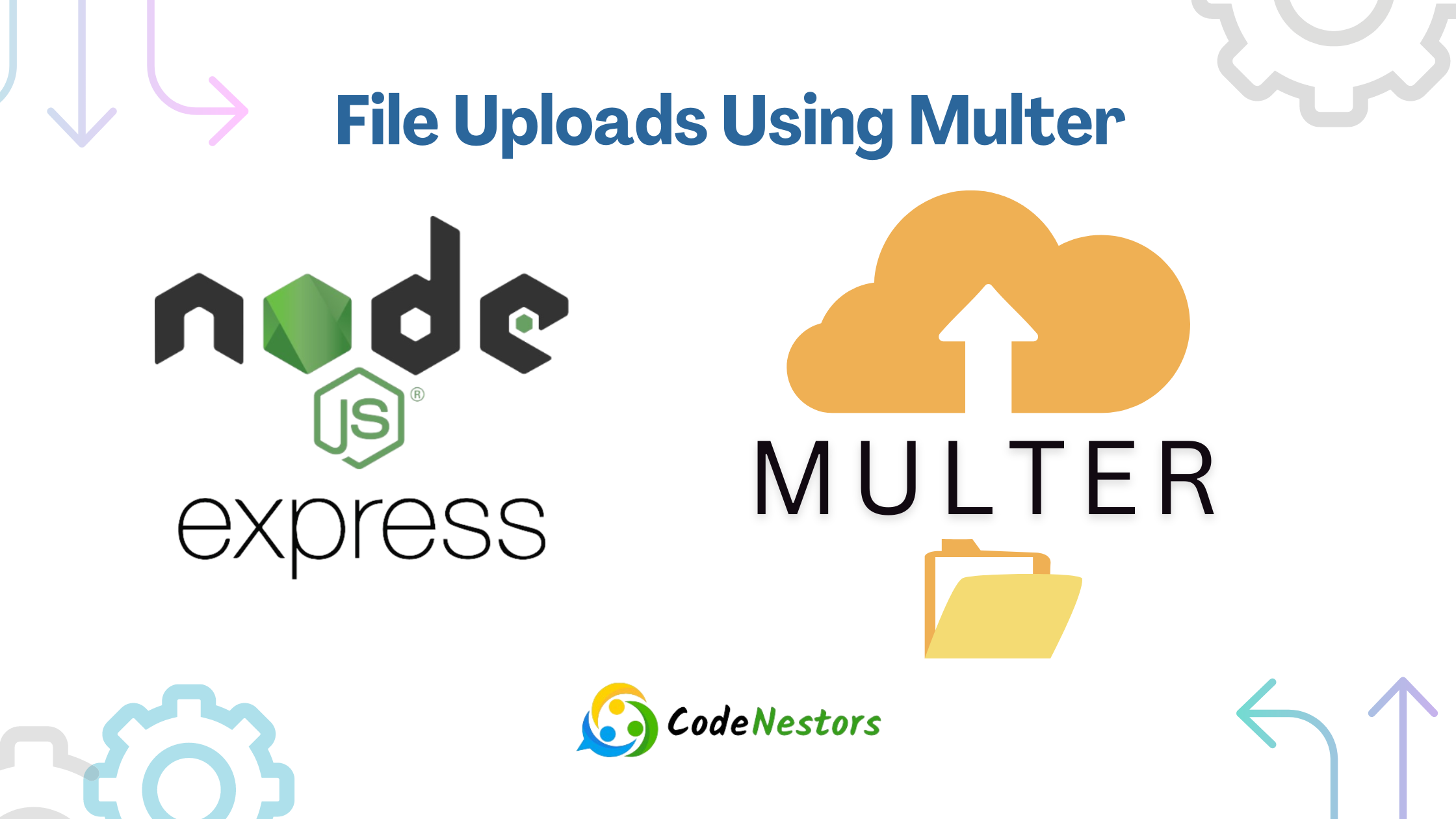
Web developers must understand how to handle file uploads in the fast-changing world of web development. Multer in Node.js is a robust solution for this task. This article explores Multer features, installation process, advanced functionalities and best practices for seamless integration with Express.
Brief Explanation of Multer in Node js
Multer is a middleware for handling multipart/form-data, which is used for uploading files in web applications. It simplifies the process of handling file uploads in Node.js making it an essential tool for developers dealing with user-generated content.
Importance of Handling File Uploads in Web Applications Express Js
Web applications often require the ability to receive files from users such as images, documents or videos. Multer streamlines this process, ensuring secure and efficient file uploads.
What is Multer?
Definition and Purpose
Multer primary purpose is to parse and handle HTTP requests with multipart/form-data
enabling developers to manage file uploads effortlessly. It provides a convenient interface for storing files on the server.
How Multer Differs from Other File Upload Modules
While various modules cater to file uploads in Node.js stands out with its simplicity, flexibility and seamless integration with Express. Unlike other modules Multer is specifically designed for handling multipart/form-data.
Installing Guide
To incorporate Multer into your Node.js project follow these simple steps:
- Open your terminal.
- Navigate to your project directory.
- Run the command:
npm install multer
Dependencies and Compatibility
Multer has minimal dependencies and is compatible with the latest versions of Node.js. It seamlessly integrates with Express enhancing the overall development experience.
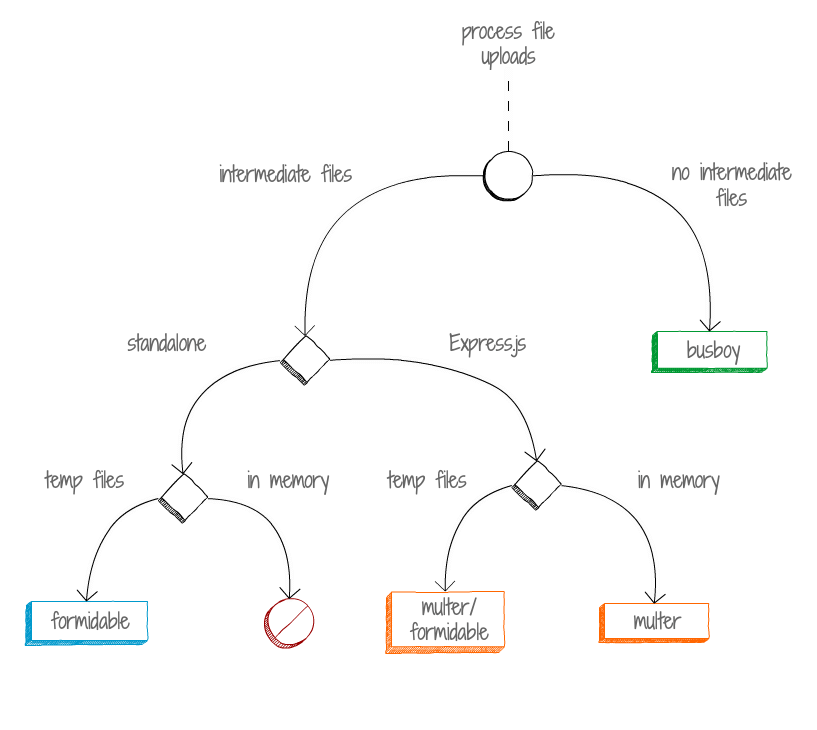
Basic Usage of Multer in Express JS
Setting Up Multer in a Node.js Project
After installation configure project by requiring it and defining the storage destination. Here’s a basic setup:
const multer = require('multer');
const storage = multer.diskStorage({
destination: function (req, file, cb) {
cb(null, 'uploads/');
},
filename: function (req, file, cb) {
cb(null, file.fieldname + '-' + Date.now());
},
});
const upload = multer({ storage: storage });
Handling Single and Multiple File Uploads with Multer node js
Multer simplifies the process of handling single and multiple file uploads. Use the upload.single('file')
middleware for a single file or upload.array('files', 3)
for multiple files, limiting to three in this example.
Configuring File Destinations and Naming Conventions
Customize the destination and naming conventions for uploaded files by modifying the storage options in the Multer setup.
File information
Key | Description | Note |
---|---|---|
fieldname | Field name specified in the form | |
originalname | Name of the file on the user’s computer | |
encoding | Encoding type of the file | |
mimetype | Mime type of the file | |
size | Size of the file in bytes | |
destination | The folder to which the file has been saved | DiskStorage |
filename | The name of the file within the destination | DiskStorage |
path | The full path to the uploaded file | DiskStorage |
buffer | A Buffer of the entire file | MemoryStorage |
Advanced Features
Handling Different File Types
Multer supports filtering file types during upload, ensuring only specified formats are accepted. Utilize the fileFilter
function to implement custom logic for file type validation.
Limiting File Size and Type
Enhance security by limiting the size and type of files that users can upload. Multer allows developers to set size limits and define accepted MIME types reducing the risk of malicious uploads.
Customizing Storage and Naming Options
Developers can tailor storage options, allowing for greater flexibility in organizing and naming uploaded files based on specific project requirements.
Integrating Multer with Express Js
Creating an Express Route for File Uploads
Integrating Multer with Express involves creating a dedicated route for file uploads. Define an endpoint and utilize Multer middleware to handle incoming files.
Implementing Error Handling and Validation
Ensure robustness by implementing error handling and validation in the Express route. Detect and address issues such as file size exceeding limits or unsupported file types.
Security Considerations in Multer
Preventing Common Security Vulnerabilities
Multer includes features that contribute to secure file uploads. Developers should validate and sanitize user inputs, preventing common security vulnerabilities such as code injection or file overwrites.
Validating and Sanitizing User Inputs
Implement input validation and sanitization to protect against potential attacks. Multer provides hooks for validating and sanitizing file names, ensuring the integrity of uploaded content.
Handling Malicious File Uploads
Developers should be vigilant against malicious file uploads. Multer configurable options, combined with proper input validation, offer robust defenses against potential security threats.
Performance Optimization of Multer
Efficiently Managing Large File Uploads
Optimize performance when handling large file uploads by configuring Multer to stream data and efficiently manage memory usage. This ensures a smooth experience for both users and the server.
Best Practices for Improving Speed and Scalability
Adopt best practices for improving the speed and scalability of file uploads. Multer’s versatility allows developers to implement optimizations based on the specific requirements of their projects.
Real-world Examples of Multer in Node js
Showcasing Multer in Practical Web Development Scenarios
Explore real-world scenarios where Multer shines. From user profile picture uploads to document attachments, Multer’s adaptability makes it a go-to choice for handling various file upload requirements.
Code Snippets and Explanations
Provide practical code snippets to showcase the simplicity and effectiveness of Multer in handling file uploads. Break down the code to help developers understand the implementation process.
Multer is a middleware for handling multipart/form-data
, which is primarily used for file uploads in Node.js. It works with the Express web framework. Below are some real-world examples of using Multer in Node.js for handling file uploads:
1. Basic File Upload
2. Handling Multiple Files
3. Limiting File SizeConclusion
In conclusion offers a powerful and flexible solution for handling file uploads. Its versatility combined with features like security considerations, performance optimization and real-world examples makes it an indispensable tool for web developers.
As you embark on your journey with Multer explore its capabilities further and implement it in your projects. The seamless integration with Express and the myriad of features make Multer a valuable asset in your web development toolkit.
FAQs
Is Multer suitable for large-scale applications with high traffic?
Multer is designed to handle file uploads efficiently, making it suitable for applications with varying traffic volumes. However, developers should implement additional optimizations for large-scale scenarios.
Can Multer be used with frameworks other than Express?
While Multer is commonly used with Express, its modular design allows integration with other frameworks. Developers may need to adapt the configuration based on the framework's specifications.
What alternatives to Multer exist for handling file uploads in Node.js?
While Multer is a popular choice, alternatives like Formidable and Busboy also cater to file uploads in Node.js. Developers can explore these options based on specific project requirements.