Mastering React Hooks: Guide to Stateful Logic, Side Effects, and Best Practices
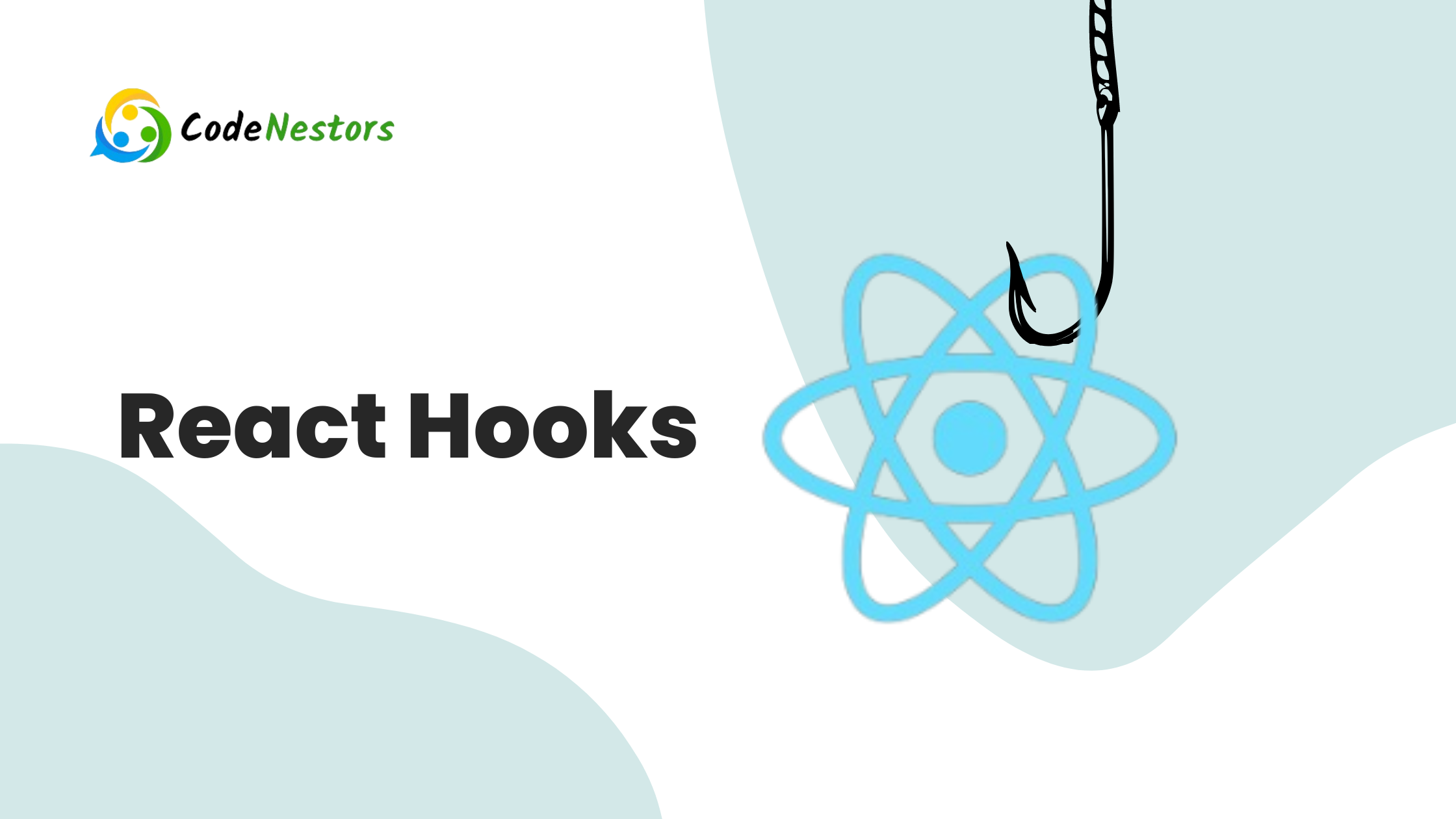
introduction
React Hooks introduced in React 16.8 revolutionized the way developers manage statefull logic in functional components. Hooks provide a cleaner and more concise way to handle side effects, state and other React features. Enabling developers to build more modular and readable code. In this article we will take a deep dive into React Hooks. Exploring their core concepts, various types and best practices for effective usage.
what is react hooks ?
A React Hook is a function that allows functional components to use state, lifecycle methods and other React features that were previously exclusive to class components. Hooks enable developers to reuse logic, manage side effects and handle state in a more modular and readable manner.
let’s explore react hooks one by one
1. useState
what is useState ?
useState is a React hook that enables functional components to manage state. Before the hooks state management primarily belonged to class components. However with the advent of useState functional components gained the ability to hold and update their own state ushering in a new era of simplicity and conciseness.
The syntax of useState is straightforward. It is a function that takes an initial state value as an argument and returns an array with two elements. first one is current state value and second one is function to update that state.
import React, { useState } from 'react';
const ExampleComponent = () => {
// Syntax: const [state, setState] = useState(initialState);
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
In this example, count is the state variable, and setCount is the function used to update the state. The initial state is set to 0.
benefits of useState hook
- Simplicity and Readability : The useState hook significantly simplifies state management resulting in more readable and concise code. With a clear syntax and a focused purpose. it eliminates the need for verbose class components.
- Improved Component Isolation : Functional components using useState are more self-contained and encapsulated. Each component can manage its own state without relying on external class-based state management.
- Seamless Integration with React Features : useState seamlessly integrates with other React features and hooks providing a cohesive and intuitive development experience. It plays well with the broader React ecosystem.
- Consistent API for State Management : Hooks introduce a consistent API for state management across functional components. Whether you’re managing simple numeric values or complex objects the pattern remains consistent.
2. useEffect
what is useEffect hook ?
useEffect is a React hook designed for handling side effects in functional components. Side effects include data fetching, subscriptions or manually changing the DOM – operations that go beyond the realm of managing component state. useEffect provides a mechanism to perform these tasks in a way that aligns with the component’s lifecycle.
The syntax of useEffect is straightforward. It takes two arguments. first one is function that contains the code to run for the side effect and second one is an optional dependency array that controls when the effect runs.
import React, { useEffect, useState } from 'react';
const ExampleComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Code to run for the side effect
// Fetch data, subscribe to something, etc.
// Cleanup function (optional)
return () => {
// Code to clean up after the component
};
}, []); // Dependency array controls when the effect runs
};
In this example the effect runs after the initial render (componentDidMount in class components) due to the empty dependency array.
benefits of useEffect hook
- Lifecycle Management : useEffect aligns with the component’s lifecycle offering equivalent functionality to componentDidMount, componentDidUpdate and componentWillUnmount in class components. This makes it intuitive for developers familiar with these lifecycle methods.
- Clean and Predictable Code : By encapsulating side effects within useEffect the code becomes cleaner and more predictable. The separation of concerns makes it easier to reason about component behavior.
- Dependency Array for Control : The optional dependency array in useEffect provides fine-grained control over when the effect runs. By specifying dependencies you can ensure that the effect runs only when necessary.
- Support for Cleanup : The ability to return a cleanup function from useEffect ensures that resources are properly released and subscriptions are unsubscribed when the component is unmounted.
3. useContext
what is useContext hook ?
useContext is a React hook designed to facilitate easy access to values stored in the React context. Context in React provides a way to pass data through the component tree without having to pass props down manually at every level. useContext allows functional components to tap into this shared state effortlessly.
The syntax of useContext is refreshingly straightforward. It takes a context object (created by the React, createContext function) as its argument and returns the current context value for that context.
import React, { useContext } from 'react';
// Create a context
const MyContext = React.createContext();
// In a component, use useContext to access the context value
const ExampleComponent = () => {
const contextValue = useContext(MyContext);
// Now, contextValue contains the current value from the context
};
In this example the LanguageProvider manages the current language and the useLanguage hook allows components to seamlessly access and change the language.
benefits of useContext hook
- Simplifies Prop Drilling : useContext elegantly solves the prop drilling problem by providing a direct way for components to access shared values stored in the context. This leads to cleaner and more maintainable code.
- Centralizes State Logic : Centralizing state logic in a context provider allows for a single source of truth. This not only improves the organization of your code but also simplifies state management.
- Encourages Reusability : By encapsulating context-related logic in a provider you can easily reuse this logic across different parts of your application. The useContext hook ensures that components can effortlessly tap into this shared functionality.
4. useReducer
what is useReducre hook ?
useReducer is a React hook designed for managing state in functional components that involve complex logic. Unlike useState which is ideal for independent state variables. useReducer is particularly powerful when dealing with state transitions that depend on the previous state or involve multiple sub-values.
The syntax of useReducer involves creating a reducer function that specifies how the state should be updated based on different actions. It returns an array with the current state and a dispatch function. which is used to trigger state transitions.
import React, { useReducer } from 'react';
// Reducer function
const reducer = (state, action) => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
};
// Initial state
const initialState = { count: 0 };
// Component using useReducer
const Counter = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
};
In this example reducer is the function that describes how the state should change in response to different actions and initialState sets the initial state of the component.
benefits of useReducer hook
- Improved State Management for Complex Logic : useReducer excels in scenarios where state transitions involve complex logic or depend on the previous state. It provides a structured approach to state management. there is some library like redux toolkit, Zustand, Hookstate for making state management easy for developers
- Centralized Logic with Reducers : Reducers allow for the centralization of state-related logic. This leads to more maintainable code as the logic is consolidated in one place rather than scattered throughout the component.
- Enhanced Predictability : By following the principles of immutability and clearly defining state transitions in reducers. useReducer enhances the predictability of state changes in your application.
- Better Handling of Interrelated State : In scenarios where state values are interrelated. useReducer simplifies the process by providing a mechanism to update multiple parts of the state atomically.
5. useMemo
what is useMemo hook ?
useMemo is a React hook that aids in memoization a technique to optimize and store the results of expensive function calls. Memoization ensures that the computation is performed only when necessary. preventing redundant calculations and enhancing the overall performance of the application.
The syntax of useMemo involves providing a function and an array of dependencies. The function contains the logic that should be memoized and the dependencies determine when the memoized value should be recalculated.
import React, { useMemo } from 'react';
const ExampleComponent = ({ data }) => {
// Memoized value based on 'data' dependency
const memoizedValue = useMemo(() => expensiveCalculation(data), [data]);
// Now, 'memoizedValue' contains the optimized result of 'expensiveCalculation'
};
In this example the expensiveCalculation function is memoized based on the data dependency.
benefits of useMemo hook
- Performance Optimization : The primary benefit of useMemo is performance optimization. By memoizing the result of expensive computations. you prevent unnecessary recalculations. leading to more efficient and responsive components.
- Reduction of Redundant Renders : useMemo helps in reducing unnecessary renders by ensuring that a memoized value is recalculated only when its dependencies change. This leads to a more streamlined rendering process.
- Improved Responsiveness : Components utilizing useMemo often exhibit improved responsiveness. especially in scenarios involving complex calculations or data manipulations. Users experience smoother interactions due to optimized rendering.
- Memoization for Function Components : Unlike class components function components don’t have a built-in mechanism for memoization. useMemo fills this gap by providing a way to memoize values in a function component bringing the benefits of memoization to the modern React development.
6. useCallback
what is useCallback ?
useCallback is a React hook that memoizes callback functions. Memoization ensures that the callback function is not recreated on every render unless its dependencies change. This is particularly beneficial in scenarios where callback functions are passed down to child components. preventing unnecessary re-renders of those child components.
The syntax of useCallback involves providing a callback function and an array of dependencies. The callback function is memoized based on the dependencies ensuring that it remains consistent across renders unless the dependencies change.
import React, { useCallback } from 'react';
const ExampleComponent = ({ onClick, data }) => {
// Memoized callback function based on 'onClick' and 'data' dependencies
const memoizedCallback = useCallback(() => onClick(data), [onClick, data]);
// Now, 'memoizedCallback' remains stable unless 'onClick' or 'data' changes
};
In this example the onClick callback function is memoized based on the onClick and data dependencies.
benefits of useCallback hook
- Enhanced Performance : The primary benefit of useCallback is enhanced performance by memoizing callback functions. This prevents unnecessary re-creations of functions and ensures that the same function reference is used across renders when dependencies don’t change.
- Prevention of Unwanted Child Component Renders : useCallback helps in preventing unnecessary re-renders of child components by ensuring that the memoized callback passed as a prop remains consistent unless its dependencies change.
- Reference Stability : Memoizing callback functions with useCallback provides reference stability. which can be crucial in scenarios where reference equality is used for performance optimizations such as in React.memo or custom hooks.
- Controlled Recalculation of Functions : By specifying dependencies in the dependency array developers can control when the callback function should be recalculated. This fine-grained control helps in managing the memoization process.
7. useRef
what is useRef hook ?
useRef is a React hook that provides a mutable object called a “ref object.” This ref object has a .current property that can hold any mutable value. Unlike state variables changes to the .current property do not trigger a re-render. useRef is commonly used to access and interact with DOM elements. manage mutable values and persist data across renders.
The syntax of useRef involves creating a ref object using the useRef hook. This ref object can then be assigned to the ref attribute of a React element or used to hold mutable values.
import React, { useRef, useEffect } from 'react';
const ExampleComponent = () => {
// Creating a ref object
const myRef = useRef();
// Assigning the ref object to a DOM element
return <div ref={myRef}>Hello, useRef!</div>;
};
In this example, myRef is a ref object that can be assigned to a DOM element.
benefits of useRef hook ?
- Non-Reactive Updates : Changes to the .current property of a ref object do not trigger re-renders. This makes useRef suitable for managing mutable values without impacting the rendering performance.
- DOM Manipulation : useRef is a powerful tool for interacting with the DOM. It allows components to access and modify DOM elements. making it essential for tasks like focusing on input fields, measuring elements or triggering imperative animations.
- Value Persistence : Ref objects created with useRef persist across renders. This makes them suitable for scenarios where you need to store and access values consistently without causing re-renders.
- Efficient Comparison : Storing and accessing the previous values of props or state variables with useRef enables efficient comparison within components. This can be useful for implementing optimizations or tracking changes.
- Versatility : The versatility of useRef extends beyond DOM interactions. It serves as a flexible tool for various scenarios from managing mutable values to persisting data without causing re-renders.
conclusion
In conclusion React Hooks have ushered in a paradigm shift in the way developers handle stateful logic in functional components. Offering a cleaner and more concise approach to managing side effects, state and other React features, Hooks have become an integral part of modern React development. In this comprehensive exploration. we delved into various React Hooks understanding their core concepts and uncovering best practices for effective usage.
In adopting React Hooks developers can harness a powerful set of tools that not only simplify code but also enhance the modularity, readability and performance of their applications. Each hook serves a unique purpose. contributing to the overall improvement of the React development experience. As we continue to embrace this revolutionary approach the journey into crafting robust and efficient React applications becomes even more exciting.
What are React Hooks?
React Hooks are functions introduced in React 16.8 to manage stateful logic in functional components. They provide a cleaner and more concise way to handle side effects, state and other React features.
Why should I use React Hooks?
React Hooks simplify state management in functional components. eliminate the need for verbose class components and provide a modular and readable way to handle various aspects of React development.
Which React Hook is used for managing component state?
useState is the React Hook used for managing state in functional components. It allows components to hold and update their own state, bringing simplicity and conciseness to state management.
How does the useEffect hook work?
useEffect is used for handling side effects in functional components. It takes a function containing code to run for the side effect and an optional dependency array that controls when the effect runs.
What is the purpose of the useContext hook?
The useContext hook facilitates easy access to values stored in React context. It eliminates the need for prop drilling by allowing components to tap into shared state effortlessly.